Preface
Introduction
The Salespoint Framework is intended to minimize developing effort of point-of-sale applications. Salespoint 2010 users complainted about complexity, missing features and bugs. Thus, the decision was made to re-design and re-implement the framework from scratch. In 2013 some parts again were re-implemented with components of the Spring framework. Our development goal was an easy-to-use framework primarily targeted for educational purposes. As such Salespoint 6 is not tailored to any specific application, but designed with a wide area of applications in mind.
Models and design patterns employed in Salespoint 6 are inspired by Enterprise Patterns and MDA [epam]. An overview of the functionality of the new features in Salespoint 6 are detailed in this document. We would like to thank all Salespoint users who submitted their feedback and encourage future users of Salespoint 6 to do the same.
Prerequisites
Working with Salespoint requires some basic knowledge of the underlying technologies such as the Spring Framework as well as JPA to implement the persistence layer. This script gives you an introduction into these technologies. Also the Guestbook sample project is a good starting point to get familiar with the technology stack.
The Videoshop sample project
To give you an example of what applications built on top of Salespoint can actually look like, we provide the Videoshop sample project that covers a few key areas:
-
Using and extending the business modules provided by Salespoint to abstract a concrete problem domain.
-
Implementing a web layer (controllers, views) on top of Java based business services of that business domain.
-
Adding the necessary bits and pieces for technical aspects such as security and transactions.
The following sections will refer to code of the sample project here and there so it might be useful to have the repository cloned and the project imported into your IDE of choice to quickly be able to navigate these. |
What’s new in Salespoint 7.1?
Upgrade to Spring Boot 2.1 GA
#225 — Simple maintenance upgrade as 7.0 still referred to a milestone release of Spring Boot 2.0.
Allow login via email address
#222 — Added the ability to use a user’s email address for login purposes. For more details, see this section.
Improvements in the order aggregate and event handling
#226 — We added the ability to register ChargeLine
instances for a dedicated OrderLine
.
Read more about that in the updated section of Order management.
New order cancelled event and fixes in event handling in general
#230 — The creation of a PaymentAccountancyEntry
has been properly attached to the OrderPaid
event now.
An OrderCancelled
event is now published on order cancellation which triggers a rollback of the inventory updates as well as a compensating ProductPaymentEntry
.
Read more on that in the updated section The Order lifecycle.
What’s new in Salespoint 6.1?
Upgrade to Spring Boot 1.2 and Spring 4.1
For Salespoint 6.1 we upgraded to Spring Boot 1.2, which transitively updates a few third party dependencies, most notably Spring 4.1. Read more on the new features of Spring Boot in its reference documentation and skim through the upgrade notes as well. The new features of Spring 4.1 are described here.
New @EnableSalespoint annotation to simplify configuration
Activating Salespoint in a web application has been quite cumbersome so far and required a dedicated set of annotations and imports of configuration classes. To simplify this, Salespoint 6.1 introduces @EnableSalespoint
to take care of the previously manual steps. For more information on what gets acivated and imported, see the JavaDoc of the annotation.
Usage of the mail autoconfiguration shipping with Spring Boot 1.2
As Salespoint 6.1 upgrades to Spring Boot 1.2 we now use the email auto-configuration settings shipping with that release. This most notably means that you slightly have to alter your properties to configure the mail user, password and host. See Boot’s reference documentation and the list of current application properties for details.
Technical Architecture
Technically Salespoint is a library to build Java based Point of Sales (PoS) applications. Whiles these applications are usually implemented as web applications, large parts of Salespoint are also usable if the application architecture of choice is a rich client implemented in Java.
The technical foundation for Salespoint is the Spring Framework[1] and Spring Boot (as some kind of opinionated layer on top of Spring Framework to ease its applicance to a large degree. Salespoint is built using the Maven build system[2]. The reference documentation and static website are built with Asciidoctor[3].
Here you can find the salespoint class diagram about the basic structure.
1. Core concepts
Before we dive into the technical details of Salespoint, let’s make sure we understand some core concepts that will help us understand the different modules of the framework a bit better. Each of the modules consists of a bit of code and the classes can usually be grouped into 3 different buckets: entities and value objects, summarized to newables, repositories and services, summarized to injectables as well as Spring container configuration and extension code. The following sections will cast some light on these kinds of types and their special traits.
1.1. Repositories, services and controllers
Repositories and services are used ot interact with entities and value objects. Repositories usually abstract data access into an interface that simulates a collection of entities that instances can be added to, or looked up using a parameterized criteria like "all users with a certain lastname like…". As Salespoint is using a relational database as persistence backend it leverages the Java Persistence API (JPA) and Spring Data JPA to implement repositories.
As repositories abstract a collection of an aggregate root (e.g. an order with it’s charge and line items), we usually need a higher level abstraction that exposes a more use-case oriented API: a service. It fulfils a more coarse grained set of operations that might include the interaction with multiple repositories, trigger other services in the system etc.
Repositories, services and controllers are types whose instances are managed by the Spring container. Thus they’re never instantiated manually in the application code, except for unit testing purposes. Application code uses dependency injection to access
2. Maven
Maven simplifies the management of project dependencies, furthermore it downloads and organizes all dependencies. Another focus of Maven is the management of a project’s build. All settings are placed in the pom.xml
file (http://maven.apache.org/pom.html).
3. Spring
In contrast to earlier versions of the Salespoint Framework, Salespoint 6 obeys the MVC pattern. Salespoint 6 mostly provides the application services to interact with the the model of an MVC application as no parts of the view or the controller are implemented in the framework.
Salespoint 6 is designed as foundation for development of web applications, using the Spring Framework to implement views, controllers and business services abstracting the domain your application-to-be-created is applied to. To ease this development, Salespoint includes a few extensions to the Spring Framework that will avoid you having to implement very tedious tasks over and over again. Read more about this in Spring 4.
As a big new approach in development with JPA, the Spring Framework with its repository interfaces can make the work very fast, clean and easy. The Crudrepository provides the basic methods for working with the database (CRUD stands for Create, Read, Update and Delete).
4. Configuration of Salespoint 7.1.0.RELEASE
The configuration for an application can be modified on the application class in the root package (e.g. videoshop.Videoshop for the videoshop project). Methods, annotated with @Configuration
, will be scanned at the beginning of the deployment on the application server (in this case Spring Boot). This configuration files will tell the application server the settings for the application. By overriding the configuration method, you can specify the login behaviour or security functions. For a basic login strategy the videoshop is a good start. There you can see, that with authorizeRequests() an authorization will be set. Following by matchers, you can specify the pages, this authorization is made on. Further, you can easily set an login page with formLogin() and the path to the login page with loginProcessingUrl("/login"). Analogue the logout settings works like login system.
Technical Stack
7. Spring Data JPA
Spring module to easily build data acess layers using JPA 2.1 (Java Persistence API).
9. Java Money
With Salespoint 6 Money
class and its related components were replaced by the Java Money library. Prices and other monetary values are represented as org.javamoney.moneta.Money
objects. Due to the fact that all representing objects are immutable, all arithmetic functions produce a new instance.
Money first = Money.parse(“USD 23.07”);
Money second = Money.parse(“USD 18.07”);
Money sum = first.plus(second);
When used in domain objects, Money
instances need to be mapped using @Lob
.
Business modules
Salespoint ships with a set of business modules that interact with each other. The following component diagram shows the overall structure of the setup. The relationships are used as follows:
-
uses — The module has a Spring component dependency and actively interacts with the target module. Implies a type dependency into the target module as well.
-
listens to — The module contains an event listener for events the target modules publishes. Implies a type dependency into the target module as well.
-
depends on — The module has a general type dependency to the target module, i.e. it uses the target module as library.
10. User accounts
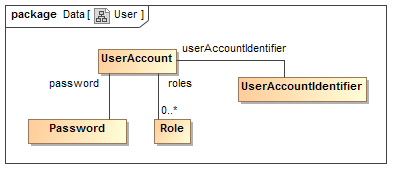
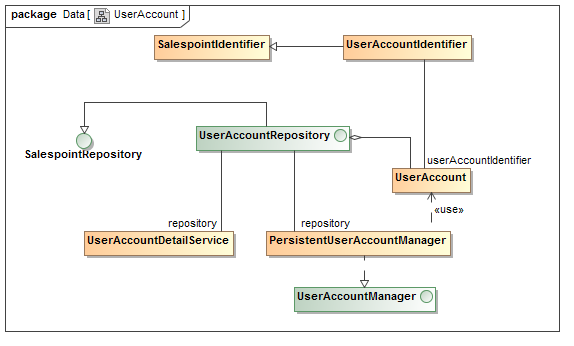
While Spring Security is used as primary technology to actually implement application security, there’s also a domain abstraction of a user acoount in the form of a UserAccount
entity.
That means that UserAccount
instances created within the system are automatically targets for authentication.
The primary purpose of a UserAccount
besides the security integration is the ability to assign other business entities like an order or a reservation to an account.
A UserAccount
primarily consists of a UserAccountIdentifier
whose String
representation is used as the login name and a Password
(representing an encrypted or non-encrypted password) value object.
To create UserAccounts
, the `UserAccountManager’s method `create(String userName, String password, Role… role)
can be used.
The String
given as password will be encrypted using Spring Security.
Also, it makes sure that the login names are unique and a password is always set.
10.1. Roles
As the name suggests, a Role
represents the role a UserAccount
has. It is usually used in security expressions with either @Secured
or in a Thymeleaf template to restrict access to functionality or change the appearance of a view depending on a user’s status.
A view for a user having an administrator role may display different content — e.g. delete buttons — than for a user not having that role.
Role
is a value type[4] and thus instances are created using a factory method: Role.of("ROLE_ADMIN");
.
10.2. Login
To reduce code repetition, Salespoint 6 contains code to automate the user log in. Using a Thymeleaf template, a special login form is generated, which is handled by an interceptor. The interceptor verifies the user password and associates the current session with the user using <login>
and <logoff>
. The session is required, because multiple users can be logged on at the same time.
As of version 7.1, Salespoint allows the login via a user’s registered email address.
To enable that, set salespoint.authentication.login-via-email
to true
.
application.properties
)salespoint.authentication.login-via-email=true
If the option is activated, UserAccountManager.save(…)
will reject UserAccount
instances that don’t have an email address set.
That means you need to provide an email when initially registering, i.e. you’ll need to use the overload of UserAccountManager.create(…)
that takes an email address as parameter.
10.3. Limitation
The org.salespointframework.useraccount.UserAccount
is limited to the given attributes and methods.
Due to the fact, that Salespoint use the SecurityContext
for authentication, the UserAccount
cannot be extended. In the background the org.salespointframework.useraccount.UserAccount
is converted to an instance of org.springframework.security.core.userdetails.UserDetails
.
If these properties don’t meet all requirements, wrap the UserAccount
in a new entity. Put all the new features in this new entity and connect this information via a @OneToOne
relationship with the UserAccount
. An example can be found in the Videoshop project.
@Entity
public class UserAccountExtension {
private String string;
private Integer integer;
@OneToOne
private UserAccount userAccount;
…
}
11. Quantity
Quantity
is used to represent amounts of anything. Furthermore a Quantity
can be used to calc with (plus, minus).
But only Quantities
with the same Metric
can be combined or compared, so every Quantity
has an Metric
attribute.
And of course, every Quantity
has an amount value, represented as a java.math.BigDecimal
.
11.1. Metric
The composite type Metric
contains all information pertaining to the unit or metric of the represented object.
Furthermore, an object of type Metric
has a description field, to explain the meaning of the metric in detail. For the example of a meter a possible description could be "The meter is the length of the path travelled by light in vacuum during a time interval of 1/299 792 458 of a second.".
12. Catalog
Salespoint 6 is intended as framework for point-of-sale applications. Items for sale are called products and represented by instances of the class Product
. To represent different kinds of products, Product
can be sub-classed. Products are managed by a Catalog
implementation (see below). Products are identified using a ProductIndentifier
.
The Catalog
interface was designed to manage Product
instances in the system. It provides functionality to add, remove, and find products. Products can be searched by their name or category. The PersistentCatalog
is an implementation of the Catalog
interface.
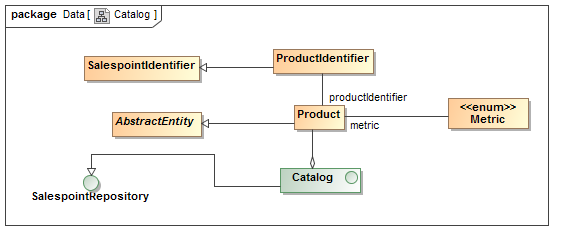
13. Inventory
The inventory package contains functionality to keep track of the amount of items we have for a given Product
(see Catalog for details).
Inventory
is a simple Spring Data repository, that allows to access InventoryItem
instances:
public interface Inventory<T extends InventoryItem> extends CrudRepository<T, InventoryItemIdentifier> {
/**
* Returns all {@link InventoryItem}s that are out of stock (i.e. the {@link Quantity}'s amount is equal or less than
* zero).
*
* @return will never be {@literal null}.
*/
@Query("select i from InventoryItem i where i.quantity.amount <= 0")
Streamable<T> findItemsOutOfStock();
/**
* Returns the {@link InventoryItem} for the {@link Product} with the given identifier.
*
* @param productIdentifier must not be {@literal null}.
* @return will never be {@literal null}.
*/
@Query("select i from InventoryItem i where i.product.id = ?1")
Optional<T> findByProductIdentifier(ProductIdentifier productIdentifier);
/**
* Returns the {@link InventoryItem} for the given {@link Product}.
*
* @param product must not be {@literal null}.
* @return will never be {@literal null}.
*/
default Optional<T> findByProduct(Product product) {
return findByProductIdentifier(product.getId());
}
}
13.1. Handling order completion events
The inventory ships with a listener for OrderCompleted
events (see The Order lifecycle for details) to update the stock for each line item contained in an Order
.
By default, an OrderCompletionFailure
is thrown in case any of the OrderLine
items aren’t available in the required amount.
The exception contains an OrderCompletionReport
that can be inspected for the item lacking enough stock.
By default, all OrderLine
instances are processed.
However, you might want to exclude some of them as the Product
they point to don’t represent a product to keep track of (e.g. some service like "gift wrapping" or the like.
The LineItemFilter
interface allows defining a strategy to decide which of the OrderLine
instances are supposed to be processed by the inventory.
To customize the strategy, simply declare an implementation of LineItemFilter
as Spring bean to your application configuration:
static class Config {
@Bean
LineItemFilter filter() {
return item -> !item.getProductName().startsWith("to filter:");
}
}
A declaration like this will cause only OrderLine
instances pointing to Product
instance with a matching name to be subject for reduction in the inventory.
On OrderCancelled
the Inventory
will restock if the Order
has already been completed.
See the Javadoc of the event listener for details.
14. Accountancy
The accountancy package contains functionality supporting book keeping. AccountancyEntry
is a representation of an accounting entry. Accountancy
aggregates AccountancyEntry
s. Every AccountancyEntry
is uniquely identified by an AccountancyEntryIdentifier
. AccountancyEntry
extends AbstractEntity
and serves as persistence entity, while PersistentAccountancy
implements Accountancy
and provides transparent access to the JPA layer. AccountancyEntryIdentifier
is used as primary key attribute, when entities are stored in the database.
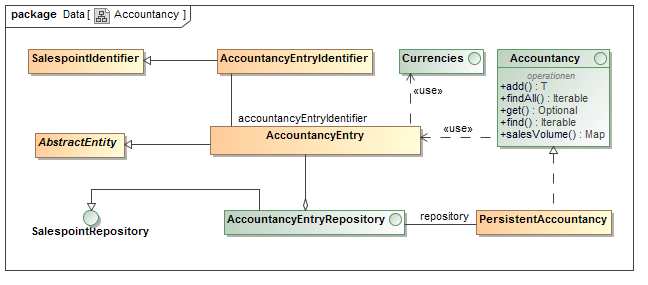
By implementing and sub-classing Accountancy
, the notion of different accounts, as known from double-entry bookkeeping, can be realized.
To create a new account, AccountancyEntry
has to be sub-classed. Every object of such a class belongs to the same account. Accessing per-account entries is facilitated by specifiying the desired class type when calling get()
or find()
methods of Accountancy
.
14.1. Handling OrderPaid events
The accountancy subsystem handles OrderPaid
events by creating a ProductPaymentEntry
for the order.
Reversely, it will create a compensating ProductPaymentEntry
on OrderCancelled
if theres a revenue ProductPaymentEntry
available for Order
.
See the Javadoc of the event listener for details.
15. Payments
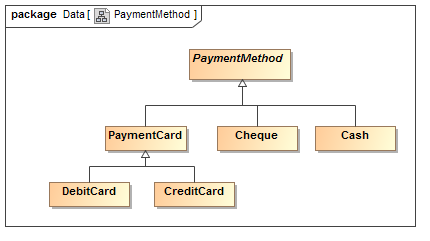
For the paying transaction, Salespoint provides some payment strategies.
-
DebitCard
— representation of e.g. EC-card or MaestroCard -
CreditCard
— equal toDebitCard
but with creditLimit-Attribute -
Cheque
— represents a written order of payment in a digital form -
Cash
— represents a payment, made direct in cash
16. Order management
The order management is centered around the Order
aggregate that aggregates OrderLine
s and ChargeLine
s.
An OrderLine
refers to a Product
from the Catalog in combination with a Quantity
.
Note, that the OrderLine
creates a copy of the Product
s description and price, so that changes to those attributes of a Product
don’t affect existing Orders
.
A ChargeLine
describes any additional charge that can be associated with the Order
, e.g. discounts, any general service charges and so on.
AttachedChargeLine
are special ChargeLine
s that are attached to an OrderLine
.
Just as a standard ChargeLine
they can be used to add charged services or discounts but attached to the particular OrderLine
instead of the overall Order
.
Order
exposes a variety of methods to lookup
16.1. The Order lifecycle
An Order
is an aggregate root[5], which means that state changes to it that have important business meaning trigger events.
These events are then used by other business modules to act on them. Currently, the following events are exposed:
-
OrderPaid
— the event being thrown if the order gets paid, usually through a call toOrderManager.pay(…)
. The Accountancy module makes use of those events as described in Handling OrderPaid events. -
OrderCompleted
— the event being thrown when theOrder
is about to be completed, usually through a call toOrderManager.completeOrder(…)
. The Inventory module ships with a listener for theOrderCompleted
event to update the inventory and reduce the stock of ordered items. See Handling order completion events for details. -
OrderCancelled
— the event being thrown when anOrder
was cancelled, usually throughOrderManager.cancelOrder(…)
. Accountancy will react by creating a newAccountancyEntry
that compensates for the revenue entry created for theOrderPaid
event. Inventory will restore the previously subtracted stock if the order had been cancelled already.
16.1.1. Handling events
In case you want to write custom code to react to those events you need to implement an event handler.
An event handler is a simple Spring component that has methods annotated with either @EventListener
or @TransactionalEventListener
.
@Component
class MyEventListener {
@EventListener
public void handleEvent(OrderCompleted event) {
// Your code goes here
}
// alternatively
@TransactionalEventListener
public void handleEventAfterTransaction(OrderCompleted event) {
// Your code goes here
}
}
The difference between the two methods is when they’re actually invoked by the framework.
The former method is called before the changes to the database are eventually persisted to the database.
That means, the event listener can throw an exception and the action that caused the event to be published in the first place will be canceled, too.
On the other hand an @EventListener
will not be guaranteed that the original action will succeed as it might be rejected if it violates e.g. database constraints.
In contrast, a @TransactionalEventListener
will be called after the original action has completed successfully.
That in turn means, that the listener has no chance to abort that action.
17. Business time
To be able to simulate the state of the system in a given point in time all components in the system that set a point in time on entities or lookup the current time use the BusinessTime
interface.
By default, the implementation will just return the current system date. However, it also allows to "forward" the current business time by calling the BusinessTime.forward(…)
method. This will augment the current business time by the duration handed into the method. E.g. forwading the business time by 2 months will return the very same time but two months ahead for each call to BusinessTime.getTime()
.
This is particularly useful for demoing purposes if e.g. orders can be timed to a certain date, credit cards become invalid after a certain point in time etc.
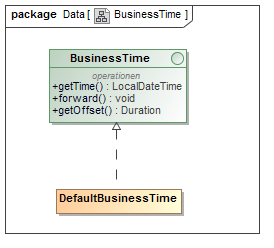
18. Technical APIs
Besides the business modules, Salespoint containes a few SPIs that can be used to extend the functionality of the core framework. These SPIs usually exist to make repeated use-cases easier to implement and free you from writing too much boilerplate code.
18.1. DataInitializer
Every application should be tested, so an easy way is, to use test data. Salespoint provides an SPI called DataInitializer
for you to implement. If your implementation is registered as Spring bean (e.g. by annotating it with @Component
), it will automatically be found and initialize()
will be called at application startup. As shown in the Videoshop project, a DataInitializer
class is registered and creates a lot of data and finally adds this data to the application.
Appendix
Appendix A: FAQ
-
Why do I see warning log statements about database tables not being accessible at startup?
This is due to a bug in Hibernate in combination with HSQLDB and constraints in databases. Hibernate tries to drop those on application startup but fails to as the (in-memory database) is empty. See this bug report for reference.
-
How do I send emails from a Salespoint application?
Salespoint has everything prepared for you in terms of necessary dependencies. All you need to do is configure the following properties in
application.properties
:spring.mail.host= spring.mail.username= spring.mail.password=
If these properties get real values assigned, Salespoint will automatically create a Spring component of type
JavaMailSender
for you that you can inject into your clients and use to send emails. See the Spring reference documentation on details for that.Never push your email credentials into the version control system. Alternatively for testing purposes, configure the
RecordingMailSender
as a Spring bean to simply write emails to be sent to the console instead of sending them to a real SMTP server. To automatically switch between the two setups have a look at how to use Spring’s profile support with Boot.
Appendix B: Glossary
- Dependency injection
-
Dependency injection is a software design pattern that implements inversion of control and allows a program design to follow the dependency inversion principle. The term was coined by Martin Fowler. See Wikipedia.
- SPI
-
Service Provider Interface — interfaces to be implemented to extend the functionality of the core framework. See Wikipedia for details.
Appendix C: Bibliography
-
[] Eric Evans. Domain-Driven Design: Tackling Complexity in the Heart of Software. Prentice Hall. 2003
-
[] Jim Arlow, Ila Neustadt. Enterprise Patterns and MDA: Building Better Software with Archetype Patterns and UML. Addison-Wesley. 2004.
-
[] Martin Fowler. Inversion of Control Containers and the Dependency Injection pattern. 2004. http://www.martinfowler.com/articles/injection.html